How to Calculate the Width of a Component in Vue.js – Complete Guide
Vue.jsPublished on , updated onLearn how to accurately calculate the width of a component in Vue.js using JavaScript, refs, and Vue’s lifecycle hooks. Step-by-step guide with examples!
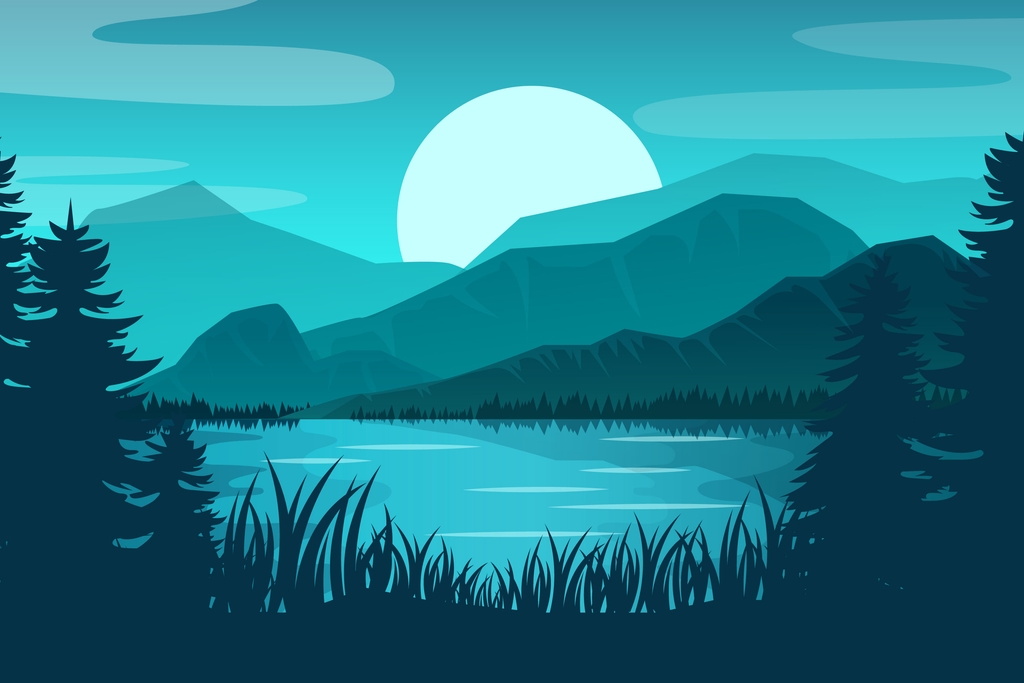
Introduction
Vue.js offers several powerful methods to determine component dimensions at runtime, allowing you to create interfaces that respond intelligently to available space rather than relying solely on CSS media queries.
This guide will walk you through everything you need to know about calculating component widths in Vue.js, from basic techniques to advanced use cases, with practical code examples you can implement in your own projects.
Understanding Component Width Fundamentals
Before diving into implementation details, it's important to understand what makes width calculation in Vue.js unique.
Unlike CSS preprocessors like SASS that calculate values at build time, Vue.js operates at runtime, giving you access to actual rendered dimensions. This distinction is crucial because it means you can:
- Respond to the actual space available to a component
- Adapt to dynamic content that might affect layout
- Create component-specific breakpoints rather than relying on viewport-based media queries
When working with Vue components, remember that dimensions like width are only accessible after the component has been rendered to the DOM. This timing consideration influences where and how you implement width calculations in your component code.
Core Methods for Calculating Component Width
Vue.js provides several approaches to determine a component's width. The two most common methods leverage Vue's direct access to DOM elements.
Using this.$el.clientWidth
The simplest approach is to access the component's root element through this.$el
:
export default {
name: 'ResponsiveComponent',
mounted() {
// Access the width of the component's root element
const componentWidth = this.$el.clientWidth
console.log('Component width:', componentWidth)
},
}
This approach works well when you need the width of the entire component. The clientWidth
property returns the inner width of an element in pixels, including padding but excluding borders, margins, and scrollbars.
Using ref
with this.$refs
For more targeted width measurements of specific elements within your component, use the ref
attribute:
<template>
<div>
<div ref="targetElement" class="element-to-measure">Content goes here</div>
</div>
</template>
<script>
export default {
mounted() {
// Access the width of the specific referenced element
const elementWidth = this.$refs.targetElement.clientWidth
console.log('Target element width:', elementWidth)
},
}
</script>
The ref
approach gives you more flexibility, especially in components with multiple elements where you need to measure specific parts independently.
Best Practices for Width Calculation Timing
When calculating width in Vue.js, timing is crucial. Attempting to measure width before a component is fully rendered can result in incorrect values or errors.
Using the mounted()
Lifecycle Hook
The mounted()
lifecycle hook is the safest place to calculate width since it guarantees that your component has been rendered to the DOM:
export default {
data() {
return {
componentWidth: 0,
}
},
mounted() {
// The component is now in the DOM and has measurable dimensions
this.componentWidth = this.$el.clientWidth
},
}
Handling Asynchronous Content
If your component loads content asynchronously (like images or data from an API), you might need to recalculate width after that content is loaded:
export default {
data() {
return {
items: [],
componentWidth: 0,
}
},
mounted() {
// Initial width calculation
this.componentWidth = this.$el.clientWidth
// Fetch data that might affect layout
fetch('/api/items')
.then((response) => response.json())
.then((data) => {
this.items = data
// Allow Vue to update the DOM
this.$nextTick(() => {
// Recalculate width after content is rendered
this.componentWidth = this.$el.clientWidth
})
})
},
}
Using $nextTick()
ensures that the DOM has been updated before you attempt to measure dimensions.
Dynamic Width Adjustment Techniques
Once you can calculate width, the next step is using that information to dynamically adjust your component.
Binding the :width
Prop
For components that accept a width property, you can bind a reactive data property:
<template>
<div>
<custom-chart :width="chartWidth"></custom-chart>
</div>
</template>
<script>
export default {
data() {
return {
chartWidth: 0,
}
},
mounted() {
this.chartWidth = this.$el.clientWidth - 40 // Subtracting padding
},
}
</script>
Using Computed Properties for Reactive Width
Computed properties provide an elegant way to derive values based on component width:
<template>
<div ref="container">
<div :class="sizeClass">This content adapts based on available width</div>
</div>
</template>
<script>
export default {
data() {
return {
containerWidth: 0,
}
},
computed: {
sizeClass() {
if (this.containerWidth < 300) return 'size-small'
if (this.containerWidth < 600) return 'size-medium'
return 'size-large'
},
},
mounted() {
this.containerWidth = this.$refs.container.clientWidth
},
}
</script>
This approach creates component-specific breakpoints that respond to the actual space available to your component, not just the viewport size.
Responsive Width Management
To create truly responsive components, you need to handle changes in available width.
Implementing Resize Event Listeners
Add a resize event listener to recalculate width when the window size changes:
export default {
data() {
return {
componentWidth: 0,
}
},
mounted() {
this.calculateWidth()
// Add resize listener
window.addEventListener('resize', this.calculateWidth)
},
beforeUnmount() {
// Clean up to prevent memory leaks
window.removeEventListener('resize', this.calculateWidth)
},
methods: {
calculateWidth() {
this.componentWidth = this.$el.clientWidth
},
},
}
For better performance, consider debouncing the resize handler:
import { debounce } from 'lodash'
export default {
data() {
return {
componentWidth: 0,
}
},
created() {
// Create a debounced version of calculateWidth
this.debouncedCalculateWidth = debounce(this.calculateWidth, 250)
},
mounted() {
this.calculateWidth()
window.addEventListener('resize', this.debouncedCalculateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.debouncedCalculateWidth)
},
methods: {
calculateWidth() {
this.componentWidth = this.$el.clientWidth
},
},
}
Advanced Scenarios
As your applications become more complex, you'll encounter more sophisticated width calculation needs.
Accessing Parent Component Width
Child components sometimes need to know the width of their parent:
export default {
data() {
return {
parentWidth: 0,
}
},
mounted() {
// Access the parent element's width
this.parentWidth = this.$el.parentElement.clientWidth
},
}
Working with Nested Components
For more complex component hierarchies, you might need to pass width information down:
<!-- Parent.vue -->
<template>
<div ref="container">
<child-component :container-width="containerWidth"></child-component>
</div>
</template>
<script>
export default {
data() {
return {
containerWidth: 0,
}
},
mounted() {
this.containerWidth = this.$refs.container.clientWidth
window.addEventListener('resize', this.updateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.updateWidth)
},
methods: {
updateWidth() {
this.containerWidth = this.$refs.container.clientWidth
},
},
}
</script>
Using the Composition API for Width Tracking
With Vue 3's Composition API, you can create reusable functionality for width tracking:
// useElementWidth.js
import { ref, onMounted, onBeforeUnmount } from 'vue'
export function useElementWidth(elementRef) {
const width = ref(0)
function updateWidth() {
if (elementRef.value) {
width.value = elementRef.value.clientWidth
}
}
onMounted(() => {
updateWidth()
window.addEventListener('resize', updateWidth)
})
onBeforeUnmount(() => {
window.removeEventListener('resize', updateWidth)
})
return { width }
}
Then use it in any component:
<template>
<div ref="container">Width: {{ width }}px</div>
</template>
<script>
import { ref } from 'vue'
import { useElementWidth } from './useElementWidth'
export default {
setup() {
const container = ref(null)
const { width } = useElementWidth(container)
return {
container,
width,
}
},
}
</script>
Practical Use Cases with Code Examples
Let's explore some real-world applications of component width calculation.
Responsive Design Adaptation
Create layouts that adapt based on available space rather than viewport size:
<template>
<div ref="container" class="card-container">
<div :class="['card-layout', layoutClass]">
<card-component
v-for="item in items"
:key="item.id"
:item="item"
:card-width="cardWidth"
></card-component>
</div>
</div>
</template>
<script>
export default {
props: {
items: Array,
},
data() {
return {
containerWidth: 0,
}
},
computed: {
layoutClass() {
if (this.containerWidth < 500) return 'single-column'
if (this.containerWidth < 800) return 'double-column'
return 'triple-column'
},
cardWidth() {
if (this.containerWidth < 500) return this.containerWidth - 40
if (this.containerWidth < 800) return this.containerWidth / 2 - 30
return this.containerWidth / 3 - 30
},
},
mounted() {
this.updateWidth()
window.addEventListener('resize', this.updateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.updateWidth)
},
methods: {
updateWidth() {
this.containerWidth = this.$refs.container.clientWidth
},
},
}
</script>
Optimized Media Loading
Load appropriately sized images based on available component space:
<template>
<div ref="imageContainer" class="image-container">
<img :src="optimizedImageUrl" alt="Responsive image" />
</div>
</template>
<script>
export default {
data() {
return {
baseImageUrl: 'https://example.com/image',
containerWidth: 0,
}
},
computed: {
optimizedImageUrl() {
// Choose image size based on container width
let size = 'small'
if (this.containerWidth > 768) size = 'medium'
if (this.containerWidth > 1200) size = 'large'
return `${this.baseImageUrl}-${size}.jpg`
},
},
mounted() {
this.containerWidth = this.$refs.imageContainer.clientWidth
window.addEventListener('resize', this.updateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.updateWidth)
},
methods: {
updateWidth() {
this.containerWidth = this.$refs.imageContainer.clientWidth
},
},
}
</script>
Performance Optimization
When working with width calculations, performance should be a consideration.
Debouncing Resize Events
As shown earlier, debouncing resize events prevents excessive calculations:
import { debounce } from 'lodash'
export default {
created() {
this.debouncedUpdateWidth = debounce(this.updateWidth, 250)
},
mounted() {
window.addEventListener('resize', this.debouncedUpdateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.debouncedUpdateWidth)
},
}
Using ResizeObserver for Targeted Monitoring
Modern browsers support ResizeObserver
, which is more efficient than window resize events:
export default {
data() {
return {
componentWidth: 0,
resizeObserver: null,
}
},
mounted() {
this.componentWidth = this.$el.clientWidth
// Create a ResizeObserver instance
this.resizeObserver = new ResizeObserver((entries) => {
for (const entry of entries) {
this.componentWidth = entry.contentRect.width
}
})
// Start observing the component
this.resizeObserver.observe(this.$el)
},
beforeUnmount() {
// Clean up
if (this.resizeObserver) {
this.resizeObserver.disconnect()
}
},
}
Accessibility Considerations
When implementing dynamic width adjustments, consider accessibility implications:
- Ensure text remains readable at all sizes
- Maintain sufficient contrast ratios when adapting colors
- Preserve focus states when layout changes
<template>
<div ref="container" class="text-container">
<p :class="textSizeClass">{{ content }}</p>
</div>
</template>
<script>
export default {
props: {
content: String,
},
data() {
return {
containerWidth: 0,
}
},
computed: {
textSizeClass() {
// Ensure text remains readable regardless of container width
if (this.containerWidth < 300) return 'text-base leading-relaxed'
if (this.containerWidth < 500) return 'text-lg leading-relaxed'
return 'text-xl leading-relaxed'
},
},
mounted() {
this.containerWidth = this.$refs.container.clientWidth
window.addEventListener('resize', this.updateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.updateWidth)
},
methods: {
updateWidth() {
this.containerWidth = this.$refs.container.clientWidth
},
},
}
</script>
Debugging and Testing
When working with component widths, proper debugging and testing are essential.
Visualizing Component Dimensions
During development, visualizing component dimensions can be helpful:
<template>
<div ref="debugComponent" class="relative">
<div class="content">
<!-- Component content -->
</div>
<div v-if="debug" class="debug-info">Width: {{ componentWidth }}px</div>
</div>
</template>
<script>
export default {
props: {
debug: {
type: Boolean,
default: false,
},
},
data() {
return {
componentWidth: 0,
}
},
mounted() {
this.componentWidth = this.$refs.debugComponent.clientWidth
window.addEventListener('resize', this.updateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.updateWidth)
},
methods: {
updateWidth() {
this.componentWidth = this.$refs.debugComponent.clientWidth
},
},
}
</script>
<style scoped>
.debug-info {
position: absolute;
top: 0;
right: 0;
background: rgba(0, 0, 0, 0.7);
color: white;
padding: 4px 8px;
font-size: 12px;
}
</style>
Testing Responsive Components
When writing tests for responsive components, you can simulate different widths:
import { mount } from '@vue/test-utils'
import ResponsiveComponent from '@/components/ResponsiveComponent.vue'
describe('ResponsiveComponent', () => {
it('applies correct class for small width', async () => {
const wrapper = mount(ResponsiveComponent)
// Mock the clientWidth value
Object.defineProperty(wrapper.vm.$refs.container, 'clientWidth', {
get: () => 250,
})
// Trigger width calculation
wrapper.vm.updateWidth()
await wrapper.vm.$nextTick()
expect(wrapper.find('.content').classes()).toContain('size-small')
})
it('applies correct class for medium width', async () => {
const wrapper = mount(ResponsiveComponent)
// Mock the clientWidth value
Object.defineProperty(wrapper.vm.$refs.container, 'clientWidth', {
get: () => 550,
})
// Trigger width calculation
wrapper.vm.updateWidth()
await wrapper.vm.$nextTick()
expect(wrapper.find('.content').classes()).toContain('size-medium')
})
})
Frequently Asked Questions (FAQs)
How do I calculate the width of a Vue.js component using a reference to the element?
Add a ref
attribute to the element in your template:
<template>
<div ref="myComponent">Content goes here</div>
</template>
Then access it in your component's methods or lifecycle hooks:
mounted() {
const width = this.$refs.myComponent.clientWidth;
console.log('Component width:', width);
}
How can I dynamically set the width of a Vue.js component?
Bind the width using a dynamic style:
<template>
<div :style="{ width: componentWidth + 'px' }">Resizable content</div>
</template>
<script>
export default {
data() {
return {
componentWidth: 300,
}
},
methods: {
resize(newWidth) {
this.componentWidth = newWidth
},
},
}
</script>
What should I consider when calculating the width of an element at runtime in Vue.js?
Remember that:
- Width is only available after the component is mounted
- Asynchronous content may change width after initial rendering
- You should clean up resize event listeners to prevent memory leaks
- Width calculations can impact performance if done too frequently
Why do I need to wait until the component is rendered to calculate its width in Vue.js?
Before the component is rendered and attached to the DOM, it doesn't have actual dimensions. Attempting to access clientWidth
or similar properties before mounting will result in incorrect values or errors. The mounted()
lifecycle hook ensures the component is in the DOM with measurable dimensions.
How can I ensure the component width is recalculated when the window size changes in Vue.js?
Add a resize event listener in the mounted()
hook and remove it in the beforeUnmount()
hook:
export default {
data() {
return {
componentWidth: 0,
}
},
mounted() {
this.updateWidth()
window.addEventListener('resize', this.updateWidth)
},
beforeUnmount() {
window.removeEventListener('resize', this.updateWidth)
},
methods: {
updateWidth() {
this.componentWidth = this.$el.clientWidth
},
},
}
Conclusion
Calculating and working with component widths in Vue.js opens up powerful possibilities for creating truly responsive, adaptive user interfaces. By understanding the core concepts and implementing the techniques covered in this guide, you can build components that:
- Adapt intelligently to available space
- Provide optimal user experiences across different devices
- Make design decisions based on component-specific dimensions rather than just viewport size
Remember these key best practices:
- Always calculate width after the component is mounted
- Clean up event listeners to prevent memory leaks
- Consider performance implications, especially in components that resize frequently
- Use appropriate APIs like ResizeObserver where available
By mastering component width calculation, you'll take your Vue.js applications to the next level of responsiveness and user experience.
This guide will demonstrate effective techniques for obtaining the width of Vue.js components. By mastering these methods, developers can ensure their applications adapt seamlessly across different devices and orientations.
Related articles
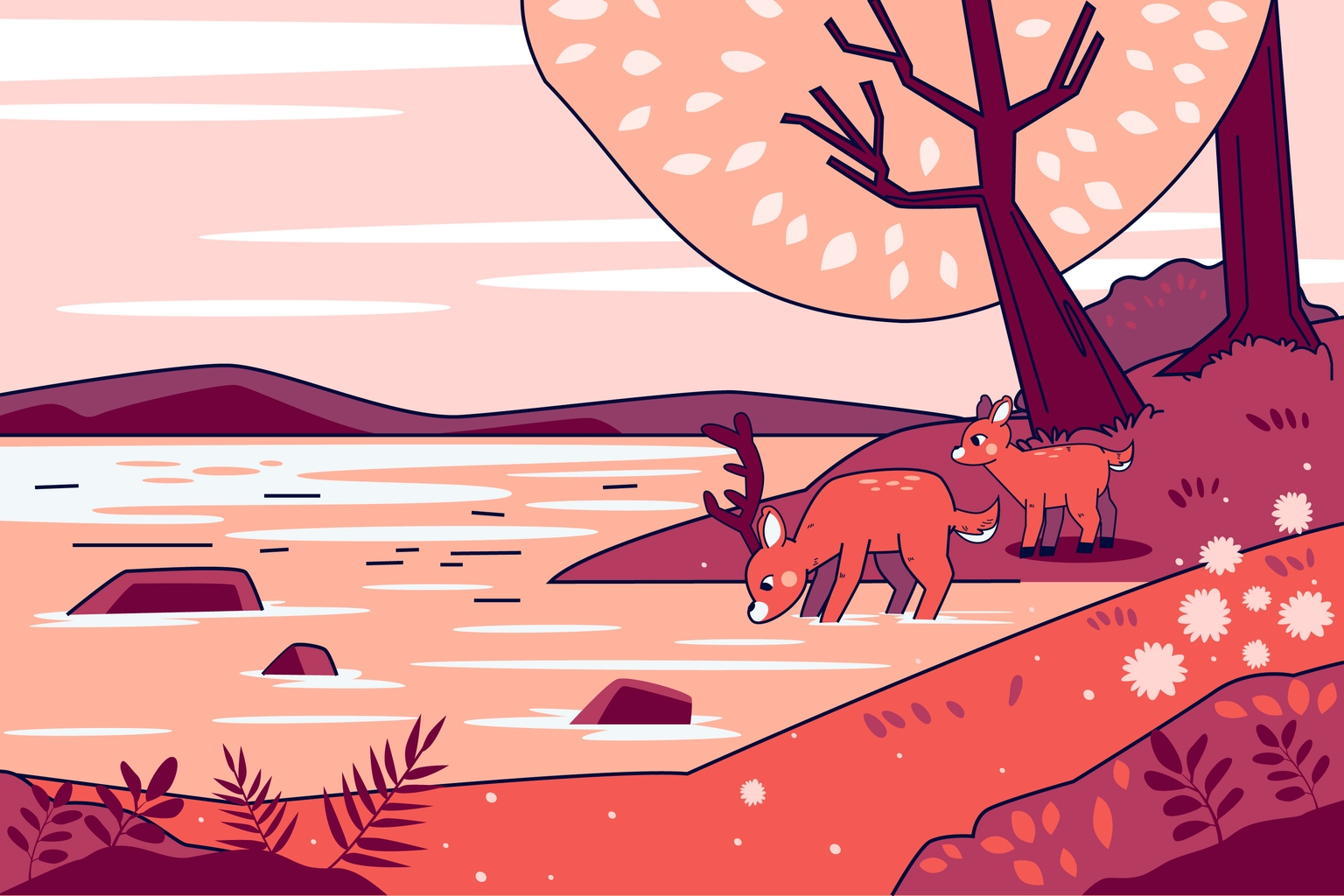
Learn how to build a multilingual blog with Nuxt Content and i18n modules. Follow this step-by-step ...